Top 25 ASP.NET Core Interview Questions and Answers
![]() |
Vivek Jaiswal |
7298 |
{{e.like}} |
{{e.dislike}} |
In this post, we will discuss the Top 25 ASP.NET Core Interview Questions and Answers. These questions are from basic to advance and a guideline for developers to prepare for the interview.
1. What is ASP.NET Core?
ASP.NET Core is a newer version of .NET. ASP.NET Core is completely rewriting that work with .net core framework. It is cross-platform, supporting Windows, MacOS and Linux, and can be used in the devices, cloud and embedded/IoT scenarios. .Net Core is compatible with .NET Framework, Xamarin and Mono, via the .Net Standard Library.
2. What is routing in ASP.NET Core?
ASP.NET Core uses a routing middleware to match the URLs of the incoming request and map them specific action methods. Using the route, routing can find route handler based on the URL.
Routing can define the routes either in startup code or as attributes. This describes how we can match the URL path with the action methods
There are two types of routing supported by ASP.NET Core
I. Conventional routing: This routing based on conventions that defined in route templates, it will map the request at runtime to controller and actions. If no route is found for the incoming request, an HTTP error of 404(Not Found) will return to the caller
Example: After creating a new project in ASP.NET Core MVC template, let’s look at the startup.cs class. We can see that the application has configured default routing in the configure method:
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
II. Attribute routing: Attribute-based routing allow the user to control the route that each controller and action take part in by using attributes that decorate to controller and actions. This approach mostly used in API.
Example:
[Route("api/User")]
[ApiController]
public class UserController : Controller
{
...
}
3. What is Metpackages?
Metapackage includes all the supported package by ASP.NET with their dependencies into one package, this introduced in .NET Core 2.0. Microsoft.AspNetCore.All is meta-package provide by ASP.NET Core. Metapackages helps developers to quick development that they don’t need to require to include the individual ASP.NET Core packages.
4. What is ConfigureServices() in ASP.NET Core
ASP.NET Core architecture mostly used Dependency Injection pattern. It includes built-in IOC container to provide a dependent object using constructors. ConfigureServices method is used to register your classes with the built-in IoC container.
After registering a dependent class, you can use anywhere in the application. You just need to include it in the parameter of the constructor of a class where you want to use it. The IoC container will inject it automatically.
Example.
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
}
5. Where to keep configuration information in ASP.NET Core.
In ASP.NET Core web.config is not available. So here we have to store configuration information in appsetting.json file, which is a plain text file, it keeps information as key-value pair in JSON format.
Example.
{
"Logging": {
"IncludeScopes": false,
"LogLevel": {
"Default": "Warning"
}
},
"Data": {
"ConnectionString": "Data Source=ADMINRG-N8EO0RN\\SQLEXPRESS;Initial Catalog=testdb;Integrated Security=True"
}
}
6. What is the startup class in ASP.NET Core?
ASP.NET Core application must include Startup class. It is same as like Global.asax in the ASP.NET webform application. This class executed first when the application starts.
The startup class can be configured using UseStartup<T>() method at the time of configuring the host in the Main() method of Program class as shown below.
public class Program
{
public static void Main(string[] args)
{
BuildWebHost(args).Run();
}
public static IWebHost BuildWebHost(string[] args)
{
WebHost.CreateDefaultBuilder(args)
.UseStartup<Startup>()
.Build();
}
}
7. What is the difference between .NET Core and .NET Framework?
Following are the main differences between .NET Core and .NET Framework
I. Cross-Platform: .NET Framework is used to develop an application for only a single platform – Windows. But .NET Core is Cross-platform, and support three distinct operating systems- Windows, OS X and Linux.
II. Open Source: .NET Framework was released as a licensed and proprietary software framework. But .NET Core released as an open-source software framework.
III. Deployment Options: .NET Framework developers have to deploy web applications only on Internet Information Server (IIS). But the web application developed in ASP.NET Core can be hosted in a number of ways like directly in cloud or self-host the application by creating their own hosting process.
IV. Application Models: The application model of the .NET Framework includes Windows Forms, ASP.NET, and Windows Presentation Foundation (WPF). On the other hand, the application model of .NET Core includes ASP.NET Core and Windows Universal Apps.
8. What is CoreCLR?
CoreCLR is the runtime for .NET Core. It includes the garbage collector, JIT Compiler, primitive data types and low-level classes.

9. How to enable session in ASP.NET Core?
The middleware for the session is provided by the package Microsoft.AspNetCore.Session. To enable session in ASP.NET Core application, we need to add this package and add the Session middleware to ASP.NET Core request pipeline (Startup.cs).
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
….
services.AddSession();
services.AddMvc();
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
….
app.UseSession();
….
}
}
10. What is Kestrel?
Kestrel is an open-source, cross-platform and default web server for ASP.NET Core. ASP.NET applications run Kestrel web server as an in-process server to handle web request. Kestrel has supported on all platforms and versions that .NET Core supports.
If your application accepts requests only from an internal network, you can use Kestrel by itself. If you expose your application to the Internet, you must use IIS, Nginx, or Apache as a reverse proxy server. A reverse proxy server receives HTTP requests from the Internet and forwards them to Kestrel. The most important reason for using a reverse proxy for edge deployments (exposed to traffic from the Internet) is security. Kestrel is relatively new and does not yet have a full complement of defences against attacks.
11. What is the purpose of IHostingEnvironment interface in ASP.NET Core?
IHostingEnvironment allow you to programmatically find the current environment so you can achieve environment-specific behaviour. ASP.NET Core has 3 environments Development, Staging and Production. You can also define your custom environments like QA and UAT.
12. What is wwwroot folder in ASP.NET Core?
The wwwroot is the root of the website. Static files can be stored in any folder under the wwwroot and accessed with a relative path to that root. Code file should be placed outside of wwwroot.
13. Dependency Injection In Asp.net Core
ASP.NET Core supports the dependency injection (DI) design pattern, It is a technique for achieving Inversion of Control (IoC) between classes and their dependencies. It allows the components in your app to have improved testability. It also makes your components only dependent on some component that can provide the needed services.
14. What are technologies discontinued in .NET Core?
Following technologies discontinued in ASP.NET Core.
I. Reflection
II. Appdomain
III. Remoting
IV. Binary serialization
V. Sandboxing
15. What are tag helpers in ASP.NET Core.?
Tag helper allows to conditionally modify or add HTML elements from server-side code. This feature available in ASP.NET Core 2.0 or later.
Examples of common built-in Tag Helpers are Anchor tag, Environment tag, Cache tag, etc.
16. What is middleware?
In ASP.NET Core Middleware controls how the application responds for Http request. It is software components that are assembled into an application pipeline to handle request and response. The incoming request passes through a pipeline where all middleware is configured, and each middleware performs some actions before passes it to the next middleware.
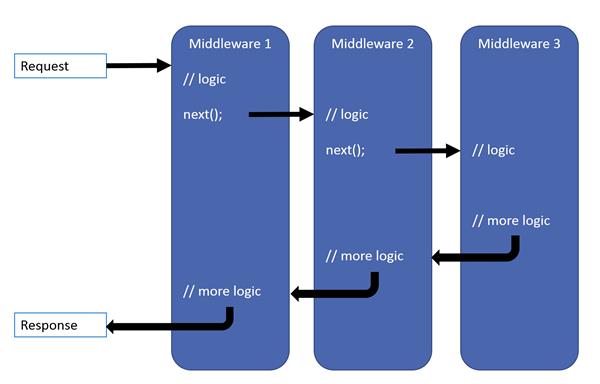
17. What is the role of WebHostBuilder() function?
The WebHostBuilder is responsible for creating the host that will bootstrap the server for the app. It is used to build up the Http pipeline via WebHostBuilder.Use() chaining it all together with WebHostBuilder.Build() by using the builder pattern. It is available in Microsoft.Aspnet.Hosting namespace.
18. How to disable Tag Helper at element level?
To disable tag helper at element level we need to use the opt-out character (“!”). This character must apply to open and close the HTML tag.
Example:
<!span asp-validation-for="email" class="txtemail"></!span>
19. What is the difference between IApplicationBuilder.Use() and IApplicationBuilder.Run()?
IApplicationBuilder.Use()
-
- It is used under the configure method of Startup class.
- It is used to add middleware delegate to the application request pipeline.
- It will call the next middleware in the pipeline.
IApplicationBuilder.Run()
-
- It is used under the configure method of Startup class.
- It is used to add middleware delegate to the application request pipeline.
- It will not call the next middleware in the pipeline. The system will stop adding middleware after this method.
20. Can ASP.NET Core work with the .NET framework?
Yes, ASP.NET Core application work with .NET framework via the .NET standard library.
21. What are the different JSON files available in ASP.NET Core?
Following are the different JSON files that used in ASP.NET Core.
-
- appsettings.json
- bundleconfig.json
- global.json
- npm.shrinkwrap.json
- launchsetting.json
- bower.json
- package.json
22. What are the advantages of ASP.NET Core over ASP.NET?
-
- NET Core is cross-platform, so you can run it on Windows, Mac and Linux.
- It can handle lot more request than ASP.NET
- You don't need to install the .NET Framework to run it. Instead, you ship all the required dlls with your application.
- You can use the Visual Studio Code to develop your application, which is free and lightweight in comparison to Visual Studio. And you can use it on different platforms as well.
23. What is Microservices in ASP.NET Core?
Microservices are the type of API services, which is small, modular and can be deployed independently. It can run in an isolated environment.
24. What is new in ASP.NET Core 2.0, compared to ASP.NET Core 1.0?
Following features added in ASP.NET Core 2.0
-
- Razor Pages.
- dotnet new now restore NuGet packages automatically.
- The configuration is now part of DI and ready for the time server reaches Startup class.
- Simplified Application Host Configuration.
- New meta-package – Microsoft.AspNetCore.All.
- DbContext Pooling with Entity Framework Core 2.
25. What is WebListener?
WebListener is a web server for ASP.NET Core that runs only on Windows. WebListener is an alternative to Kestrel that can be used for direct connection to the Internet without relying on IIS as a reverse proxy server. In fact, WebListener can't be used with IIS or IIS Express.
Thanks.
If you like, please share with your friends.
Comments
![]() |
|
Follow up comments |
{{e.Name}} {{e.Comments}} |
{{e.days}} | |
|
||
|
{{r.Name}} {{r.Comments}} |
{{r.days}} | |
|